OAuth2 With API Platform
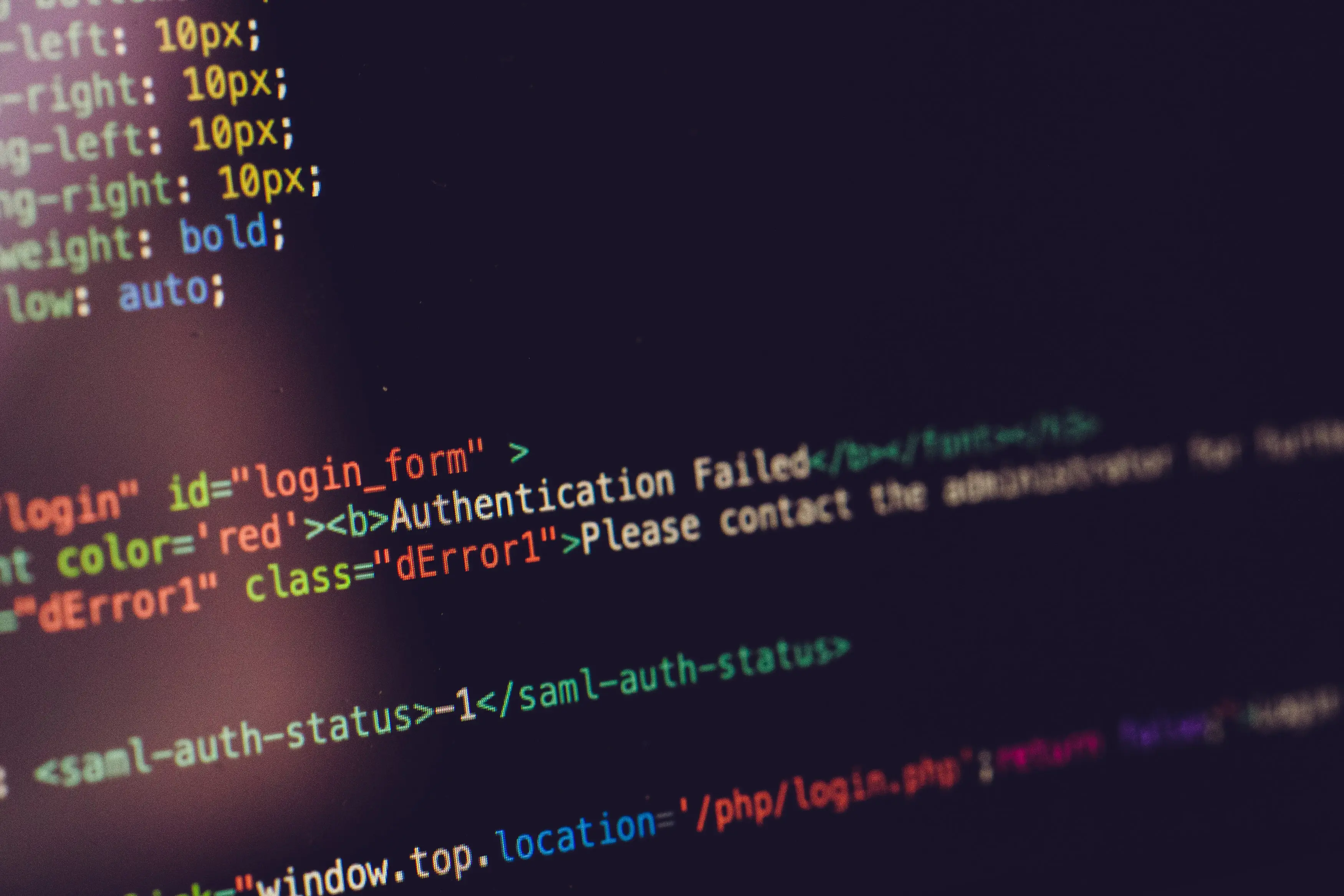
API Platform has a good JWT implementation guide which helps you implement JWT token authentication with username and password, be sure to check out the configuration page as well.
But if you also want to implement the OAuth2 workflow while also using your own authorization server, you could go ahead and use the FOSOAuthServerBundle for that.
For testing endpoint functionality, I am using Insomnia as an alternative to Postman, for example.
The crux (“simply”) lies within securing the API endpoint of your choice with fos_oauth: true
and secure your controller
functions further using the symfony built-in security or easier using
SensioFrameworkExtraBundle’s
@IsGranted('ROLE_[...]')
directive.
Check out this config/security.yml
configuration, we got the token
endpoint, the authentication
endpoint and the api
(prefix)
endpoint declared, where the /api
endpoint is secured by fos_oauth
.
(stepping through the FOSOAuthServerBundle documentation here)
security:
firewalls:
oauth_token:
pattern: ^/oauth/v2/token
security: false
oauth_authorize:
pattern: ^/oauth/v2/auth
guard:
authenticators:
- lexik_jwt_authentication.jwt_token_authenticator
api:
pattern: ^/api
fos_oauth: true
stateless: true
anonymous: false # can be omitted as its default value
access_control:
- { path: ^/api, roles: [ IS_AUTHENTICATED_FULLY ] }
At this point, create a client using the command php app/console fos:oauth-server:create-client --redirect-uri="..." --grant-type="..."
for it, use the client_id
and client_secret
for authorization at the token endpoint.
The --redirect-uri
is the ‘success’ URL after authorization, the --grant-type
depends on the client you want to create.
If you want to create a client using the
client credentials flow,
this would be client_credentials
.
And you are able to access your secured /api
endpoint.
Consider this note about security concerning flawed session management inside Symfony.
At this point there is one more problem I have not quite gotten my head around.
If you are using API Platform, you might be familiar with the OpenAPI Specification Support (former Swapper UI).
Aside the fact that you do not have a proper endpoint decorator for your login yet, you are not able to log in with that right away.
I have used KnpUOAuth2ClientBundle
with the generic
configuration which gives you the ability to authorize using your client_id
/client_secret
combination
but this did not seem to work just yet.
You are still able to test the endpoint login using Insomnia, though.
Of course the KnpUOAuth2ClientBundle enables you to login using different services if needed some time.